It provides the steps to connect AWS S3 service and create bucket, folder, upload and download files from amzon S3. Copuple of examples are shown for Java using AWS JAVA SDK library. Sample code uses aws-java-sdk-1.0.12.jar library provided by AWS. Wanted to keep the Java code short and simple.
This code connect AWS S3 service and get the list of the buckets,
creating new bucket and new folder inside the bucket. Downloading and
uploading files to the S3 folder inside a bucket. From my experience
what I noticed that people face the main challenge to coneect the S3
service from Java client. But once the able to connect, they can easy
create bucket, folder and uploading & downloading.
Java Code To Connect S3 Service:
package easycodeforall.azs;
import java.io.ByteArrayInputStream;
import java.io.InputStream;
import com.amazonaws.auth.AWSCredentials;
import com.amazonaws.auth.BasicAWSCredentials;
import com.amazonaws.services.s3.AmazonS3;
import com.amazonaws.services.s3.AmazonS3Client;
import com.amazonaws.services.s3.model.Bucket;
import com.amazonaws.services.s3.model.ObjectMetadata;
import com.amazonaws.services.s3.model.PutObjectRequest;
public class TestS3 {
public static void main(String[] args) {
String accessKeyID = "AKIA***********";// You get it form AWS Console
String secretAccessKey = "VFqXaw7KGYL****************"; // You get it form AWS Console
AWSCredentials awsCredentials = new BasicAWSCredentials(accessKeyID, secretAccessKey);
// 0. Create a S3 Client
AmazonS3 s3client = new AmazonS3Client(awsCredentials);
// 1. Get all bucket names.
s3client.listBuckets();
for (Bucket bucket : s3client.listBuckets()) {
System.out.println("bucketName=" + bucket.getName());
}
System.out.println("---------bucketName Done--------------");
// 2. create bucket
String bucketName = "demobucketxy1s";
s3client.createBucket(bucketName);
System.out.println("---------Create Bucket Done--------------");
// 3. Create a folder in a bucket.
String folderName = "testfolder";
ObjectMetadata metadata = new ObjectMetadata();
metadata.setContentLength(0);
InputStream emptyContent = new ByteArrayInputStream(new byte[0]);
PutObjectRequest putObjectRequest = new PutObjectRequest(bucketName, folderName + "/", emptyContent, metadata);
s3client.putObject(putObjectRequest);
System.out.println("---------Create Folder Done--------------");
}
}
Now question is how we can get the accessKeyID
and secretAccessKey
|
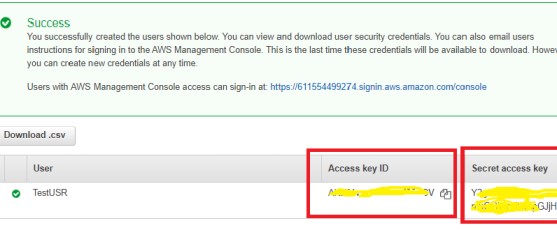 |
To have the access key Please execute the following steps
'
- Create an IAM user
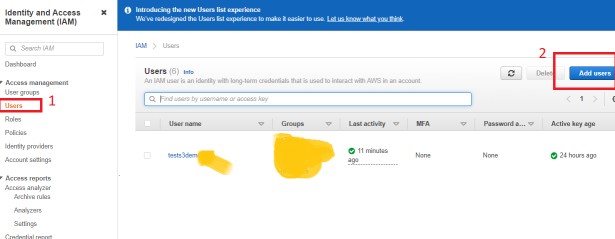
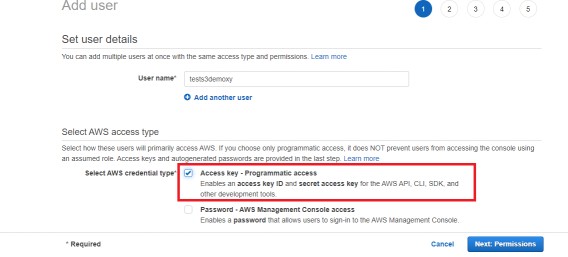
- Add permission to the user by attaching a policy. You
can create a Policy to access the S3 service and attach the policy to
the user.
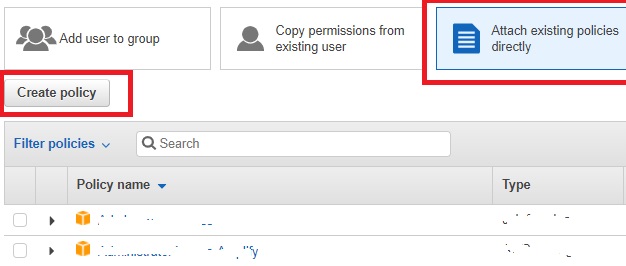
- Create a Access Key - Once you add the
successfully, it automatically create a pair of accessKeyID and
secretAccessKey .
USE THIS VALUES IN THE JAVA CODE TO
CONNECT S3 SERVICE.
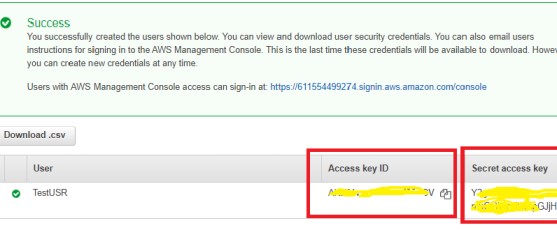
Please provide your feedback here